Authentication
You'll need to authenticate your requests to access any of the endpoints in the House Check API. In this guide, we'll look at how authentication works.
House Check offers one way to authenticate your API requests: OAuth2.
Create a client.
On our website you can configure a API client. Here you can specify the name and the redirect_uri's for your api key.
Note that you can specify multiple redirect uri's, these should be comma separated.
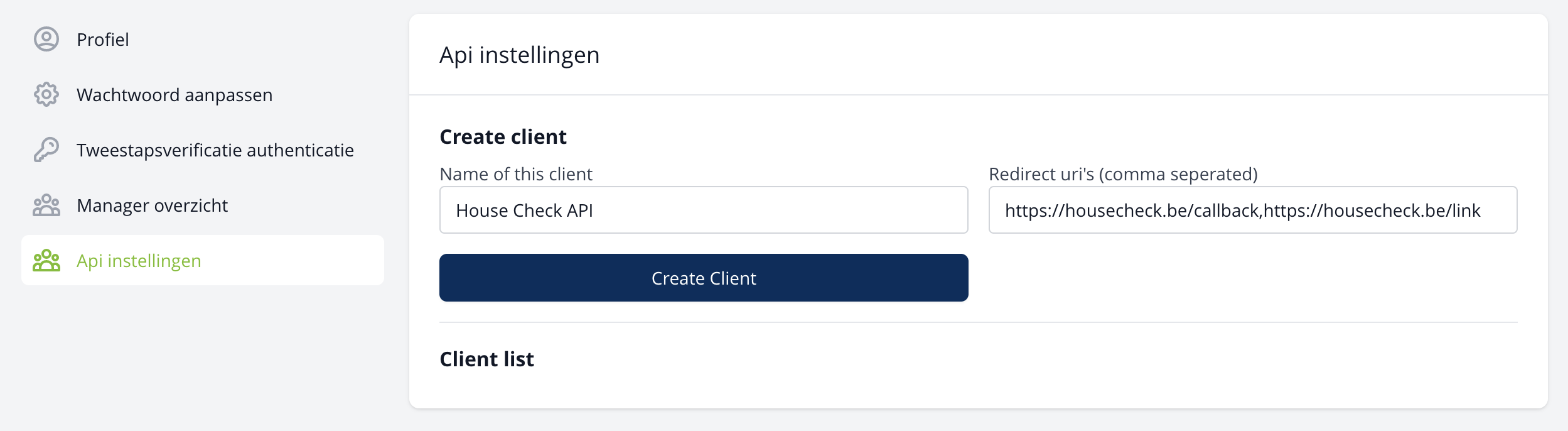
When a client is configured, you can access the client id and client secred that is used for authenicating the api as descibed below.
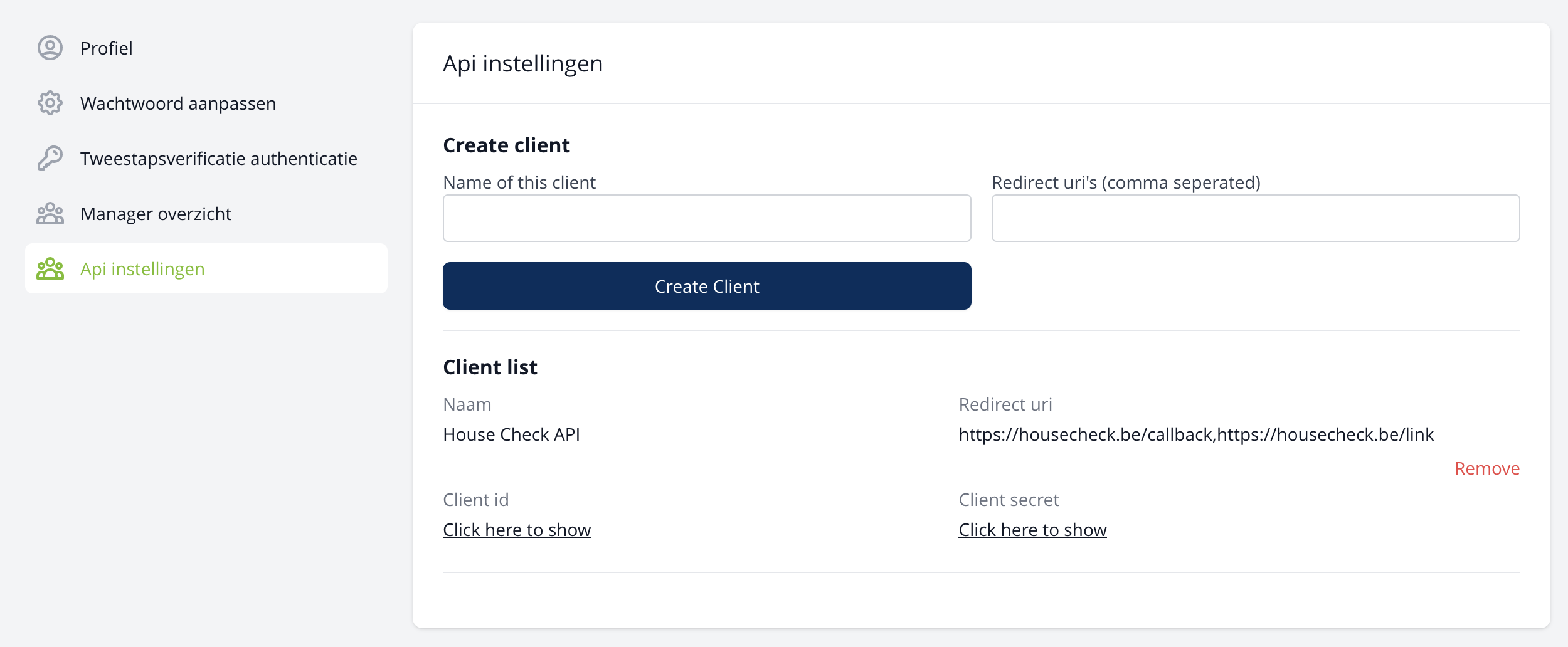
OAuth2
OAuth 2 is an authorization framework that allows a user to grant limited access to data in a House Check account, without having to expose their credentials.
To get access to the protected resources using our API, OAuth 2 uses access tokens. An access token is a string representing the granted permissions. Access tokens can be obtained after a user has completed the OAuth 2 authorization flow.
Before starting, you will need to register your integration. Each registered integration is assigned a unique client_id
and client_secret
, which is used in the OAuth 2 authorization flow. Note that the client_secret
key should not be shared or embedded in client-side code.
Always keep your token safe and reset it if you suspect it has been compromised.
Authorization flow
To get access to a user's House Check data using the authorization code
grant type, redirect users to the House Check authorization page.
Properties
- Name
client_id
- Type
- string
- Description
Issued when you create your integration.
- Name
redirect_uri
- Type
- string
- Description
URL to redirect back to after authorization.
- Name
response_type
- Type
- string
- Description
Must be
code
.
- Name
state
- Type
- string
- Description
Unique string to be passed back upon completion (optional)
A list of allowed redirect URIs needs to be configured on your integration's settings page. Only redirect URIs matching one of these whitelisted URIs will be accepted. This is a security measure to prevent malicious users to impersonate your integration.
After logging in, the user will be asked to authorize your integration to access the data in their account. You will only be granted access to certain House Check data.
If the user authorizes your integration, they will be redirected to the specified redirect_uri
with a temporary authorization code and the original state parameter. If the state parameter does not match the original value, the response may have been created by a third party and should be ignored.
https://YOUR_REDIRECT_URI?code=CODE&state=STATE
If the user denies your integration, he will be redirected to the redirect_uri, with an error parameter:
https://YOUR_REDIRECT_URI?error=access_denied
Obtaining access tokens
After receiving the authorization code from the previous step, an access token can be requested to make API calls. Note that authorization codes can only be exchanged for an access token once and expire 10 minutes after issuance. To exchange the code for an access token.
Properties
- Name
grant_type
- Type
- string
- Description
Must be
authorization_code
.
- Name
client_id
- Type
- string
- Description
Issued when you register your integration.
- Name
client_secret
- Type
- string
- Description
Issued when you register your integration.
- Name
code
- Type
- string
- Description
The authorization code.
- Name
redirect_uri
- Type
- string
- Description
The original submitted redirect URL.
Response
{
"token_type":"Bearer",
"expires_in":86400,
"access_token":"ACCESS_TOKEN",
"refresh_token":"REFRESH_TOKEN"
}
These access tokens are also known as bearer tokens. You can use this token to call API endpoints on behalf of the user, by adding it to the API request as an Authorization
header.
Access tokens expire shortly (1 day) after they are issued for security reasons. If your integration needs to communicate with our API beyond the access token's lifespan, you will need to request a new access token using the refresh token which was issued with the access token. Note that refresh tokens can only be used once to get a new access token and refresh token. The refresh token will be valid for 3 days.
Using refresh tokens
If an access token is expired, a new access token and refresh token pair can be obtained.
Properties
- Name
grant_type
- Type
- string
- Description
Must be
refresh_token
.
- Name
client_id
- Type
- string
- Description
Issued when you register your integration.
- Name
client_secret
- Type
- string
- Description
Issued when you register your integration.
- Name
refresh_token
- Type
- string
- Description
The refresh token.
User identity
To retrieve information about the user who authorized your application. (the resource owner)
Response
{
"id": 1,
"name": "John Doe",
"email": "john@doe.com",
"locale": "nl"
}