Webhooks
In this guide, we will look at how to register and consume webhooks to integrate your app with House Checm. With webhooks, your app can know when something happens in House Check, such as someone updating a order.
Webhooks are currently beeing tested, we will inform you when they go live.
Registering webhooks
To register a new webhook, you need to have a URL in your app that House Check can call. You can configure a new webhook from the House Check dashboard under website. Give your webhook a name, pick the events you want to listen for, and add your URL.
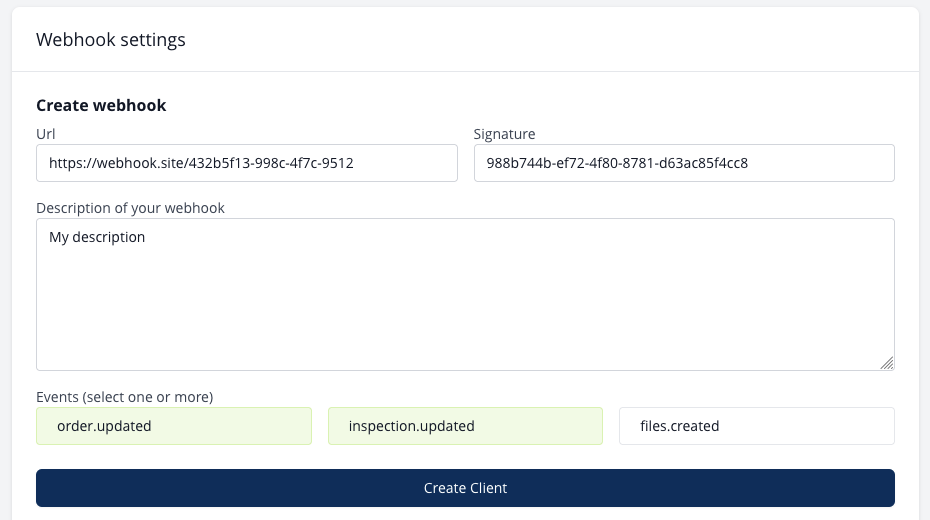
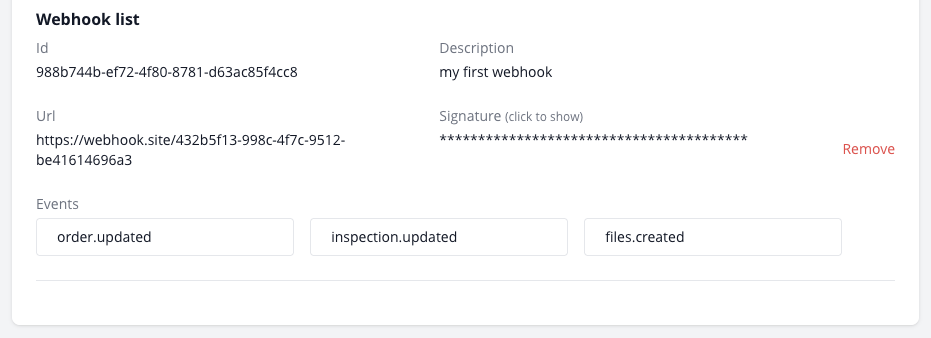
Now, whenever something of interest happens in your app, a webhook is fired off by House Check. In the next section, we'll look at how to consume webhooks.
Consuming webhooks
When your app receives a webhook request from House Check, check the type
attribute to see what event caused it. The first part of the event type will tell you the payload type, e.g., a product, order, etc.
Example webhook payload
{
"type": "order.update",
"payload": {
"id": 9001,
"status": "to schedule"
}
}
In the example above, a order was updated
, and the payload type is a order
.
Event types
- Name
order.updated
- Type
- Description
An existing order was updated.
- Name
inspection.updated
- Type
- Description
An existing inspection was updated.
- Name
files.created
- Type
- Description
One or more files are created.
Security
To know for sure that a webhook was, in fact, sent by House Check instead of a malicious actor, you can verify the request signature. Each webhook request contains a header named signature
, and you can verify this signature by using your secret webhook key. The signature is an HMAC hash of the request payload hashed using your secret key. Here is an example of how to verify the signature in your app:
Verifying a request
const signature = req.headers['signature']
const hash = crypto.createHmac('sha256', secret).update(payload).digest('hex')
if (hash === signature) {
// Request is verified
} else {
// Request could not be verified
}
If your generated signature matches the signature
header, you can be sure that the request was truly coming from House Check. It's essential to keep your secret webhook key safe — otherwise, you can no longer be sure that a given webhook was sent by House Check. Don't commit your secret webhook key to GitHub!